JavaScript Tips & Tricks
Best JavaScript tricks and tips
Published:Conditionally Add Properties to an Object
We can use the spread operator ...
to dynamically add properties to an object.
const condition = true;
const player = {
id: 1,
name: 'Super Mario',
...(condition && { age: 25 }),
};
console.log(player); // { id: 1, name: 'Super Mario', age: 25 }
The &&
operator in JavaScript returns the second operand only if the first is evaluated as true. So, if condition
is true, the player
object will be given the property { age: 25 }
. If condition
had been false, nothing would have been added.
Check if a Property Exists in an Object
We can use the in
keyword to check if a property exists in an object.
const jobAdvertisement = { company: 'google', workLocation: 'Milan' };
console.log('workLocation' in jobAdvertisement); // returns true
console.log('salary' in jobAdvertisement); // returns false
Clone Objects and Arrays
In JavaScript, cloning can be done in two ways: shallow copy and deep copy.
Shallow copy only copies the top level properties. If the original contains references to nested objects or arrays, the copy will have references to the same elements, not independent copies.
Deep copy creates a complete copy, copying all levels of properties. This means every reference to a nested object or array is recursively copied, resulting in a completely independent copy.
Shallow Copy
const person = {
name: "Walter",
job: "teacher",
};
// new object
const newPerson = { ...person }; // {name: 'Walter', job: 'teacher'}
// we can also modify or add properties
const newPersonTwo = { ...person, job: "cook" };
// {name: 'Walter', job: 'cook'}
const newPersonThree = { ...person, age: 50 };
// {name: 'Walter', job: 'teacher', age: 50}
const numbers = [1, 2, 3, 4, 5, 6, 7];
const newNumbers = [...numbers]; // [1, 2, 3, 4, 5, 6, 7];
In the above example, we use the spread operator ...
to create a shallow copy of the person
object and the numbers
array. Any modifications to the original or the copy will not affect the other.
Deep Copy
const person = {
name: "Walter",
job: "teacher",
address: {
country: "New Mexico",
city: "Albuquerque",
},
};
// method 1
const clonedPerson = JSON.parse(JSON.stringify(person));
// method 2
const clonedPersonTwo = structuredClone(person);
const arrayWithObjects = [
{ id: 1, value: 'a' },
{ id: 2, value: 'b' },
{ id: 3, value: 'c' },
];
const clonedArray = JSON.parse(JSON.stringify(arrayWithObjects));
const clonedArrayTwo = structuredClone(arrayWithObjects);
In the above example, we use JSON.parse(JSON.stringify(person))
to create a deep copy of the person
object. Alternatively, we can use structuredClone
, which is a more modern and direct method for deeply cloning objects. In both cases, every level of the object is copied, producing a completely independent copy.
Merge Arrays and Objects
In JavaScript, we can use the spread operator ...
to merge objects and arrays in a simple and readable way.
const person = {
name: "Walter",
job: "teacher",
};
const address = {
state: "New Mexico",
city: "Albuquerque",
};
// Merge two objects
const personAddress = { ...person, ...address };
console.log(personAddress);
// Output: {name: 'Walter', job: 'teacher', state: 'New Mexico', city: 'Albuquerque'}
const numbers = [1, 2, 3, 4, 5, 6, 7];
const someNumber = [8, 9, 10];
// Merge two arrays
const newArrayNumbers = [...numbers, ...someNumber];
console.log(newArrayNumbers);
// Output: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
Use Destructuring to Extract Values from Objects
Destructuring in JavaScript allows you to extract values from objects in a simple and readable way.
const player = {
name: "Mario",
age: 25,
occupation: "plumber"
};
// Extract values using destructuring
const { name, age, occupation } = player;
console.log(name); // Mario
console.log(age); // 25
console.log(occupation); // plumber
// You can also set default values
const { name, age, occupation, lastname = "Super", isBig = true } = player;
console.log(lastname); // Super
console.log(isBig); // true
Use Destructuring to Extract Values from Arrays
Destructuring can also be used to extract values from arrays in a similar way.
const players = ["Mario", "Luigi"];
// Extract values using destructuring
const [firstPlayer, secondPlayer] = players;
console.log(firstPlayer); // Mario
console.log(secondPlayer); // Luigi
// You can also set default values
const [firstPlayer, secondPlayer, thirdPlayer = "Peach"] = players;
console.log(firstPlayer); // Mario
console.log(secondPlayer); // Luigi
console.log(thirdPlayer); // Peach
Set Dynamic Property Names for Objects
In JavaScript, you can dynamically assign names to object properties using square brackets []
.
const dynamic = 'age';
const person = {
name: 'Jack',
[dynamic]: 38
};
console.log(person); // { name: 'Jack', age: 38 }
We can use the same trick to retrieve the value of a property using a dynamic key:
const keyName = 'age';
console.log(person[keyName]); // returns 38
Pass Objects as Arguments
You can pass objects as arguments to functions to improve code readability and make parameters clearer.
const createPlayer = ({ name, age, job }) => {
// some logic
console.log(`Name: ${name}, Age: ${age}, Job: ${job}`);
}
createPlayer({
name: 'Mario',
job: 'plumber',
age: 25,
});
How to Iterate Over Objects
const person = { name: 'Jack', age: 38, job: 'doctor' };
// Using for...of and Object.entries
for (const [key, value] of Object.entries(person)) {
console.log(`Key: ${key}, Value: ${value}`);
}
// Using Object.keys and forEach
Object.keys(person).forEach(key => {
const value = person[key];
console.log(`Key: ${key}, Value: ${value}`);
});
// Using Object.entries and forEach
Object.entries(person).forEach(([key, value]) => {
console.log(`Key: ${key}, Value: ${value}`);
});
// Output of the three methods
// Key: name, Value: Jack
// Key: age, Value: 38
// Key: job, Value: doctor
Sort an Array Alphabetically
const array = ["Banana", "apple", "Cherry", "date"];
array.sort((a, b) => a.localeCompare(b));
console.log(array); // ["apple", "Banana", "Cherry", "date"]
Using Boolean to Check if a Value is True
const array = [null, 'Apple', undefined, false, true, 0];
// Filter out falsy values
const filtered = array.filter(Boolean);
console.log(filtered); // returns ['Apple', true]
// Check if at least one value is truthy
const someTrue = array.some(Boolean);
console.log(someTrue); // returns true
// Check if all values are truthy
const everyTrue = array.every(Boolean);
console.log(everyTrue); // returns false
Using map and Number for quick casting
const numbers = ["0", "123", "3.14", "-256", "6323.1431"];
const newNumbers = numbers.map(Number);
console.log(newNumbers); // [0, 123, 3.14, -256, 6323.1431];
Removing Duplicates from an Array with Set
We can use Set
to remove duplicates from an array. A Set
object in JavaScript allows you to store unique values of any type.
const numbers = [0, 0, 1, 2, 2, 3, 3, 4, 5, 6, 7, 7, 8, 8, 9];
const players = ["Mario", "Mario", "Luigi", "Luigi", "Toad"];
const uniqueNumbers = [...new Set(numbers)];
const uniquePlayers = [...new Set(players)];
console.log(uniqueNumbers); // [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
console.log(uniquePlayers); // ["Mario", "Luigi", "Toad"]
By converting an array into a Set
, duplicates are automatically removed. Using the spread operator [...new Set(array)]
, we can convert the Set
back into an array with only unique values.
Using the Nullish Coalescing Operator ??
The Nullish Coalescing Operator ??
in JavaScript is used to provide a default value when the left-hand operand is null or undefined.
const userName = null ?? "Default User";
console.log(userName); // Output: "Default User"
// If userName is null, "Default User" is assigned
const userStatus = undefined ?? "Guest";
console.log(userStatus); // Output: "Guest"
// If userStatus is undefined, "Guest" is assigned
const userAge = 0 ?? "Age not specified";
console.log(userAge); // Output: 0
// If userAge is 0, 0 is retained because 0 is a valid value (not null or undefined)
const userMessage = "" ?? "No message provided";
console.log(userMessage); // Output: ""
// If userMessage is an empty string,
// the empty string is retained because it is a valid value (not null or undefined)
Be careful when using ||
as you might get unexpected results.
The OR operator returns the first "truthy" operand it finds, which means it treats values like 0, "", false as "falsy" and replaces them with the provided default value.
const number = 0 || "new number";
console.log(number); // Output: "new number"
// 0 is considered "falsy", so it is replaced with "new number"
const string = "" || "new string";
console.log(string); // Output: "new string"
// "" is considered "falsy", so it is replaced with "new string"
Using Optional Chaining ?.
The Optional Chaining Operator ?.
allows you to safely access properties and methods of objects that might be null or undefined without throwing errors, making the code cleaner and more readable.
const user = {
name: "Alice",
address: {
city: "Wonderland",
zip: "12345"
},
greet: function() {
return "Hello!";
}
};
const cityName = user?.address?.city;
console.log(cityName); // Output: "Wonderland"
const countryName = user?.address?.country;
console.log(countryName); // Output: undefined
const greeting = user?.greet?.();
console.log(greeting); // Output: "Hello!"
const farewell = user?.farewell?.();
console.log(farewell); // Output: undefined
const users = [
{ name: "Charlie" },
null,
{ name: "Hurley" }
];
const firstUserName = users[0]?.name;
console.log(firstUserName); // Output: "Charlie"
const secondUserName = users[1]?.name;
console.log(secondUserName); // Output: undefined
// Example: Using in combination with Nullish Coalescing Operator
const settings = {
preferences: {
theme: null
}
};
const userTheme = settings?.preferences?.theme ?? "default theme";
console.log(userTheme); // Output: "default theme"
Casting to Boolean with !!
The double negation operator !!
is used to convert any value to a boolean in JavaScript. It is a quick and concise method for casting to boolean.
Falsy Values
const value1 = null;
const value2 = undefined;
const value3 = 0;
const value4 = "";
console.log(!!value1); // Output: false
console.log(!!value2); // Output: false
console.log(!!value3); // Output: false
console.log(!!value4); // Output: false
Values such as null
, undefined
, 0
, and empty strings ""
are considered falsy in JavaScript, and thus are converted to false
when applied with !!
.
Truthy Values
const value1 = 1;
const value2 = "Hello";
const value3 = [];
const value4 = {};
console.log(!!value1); // Output: true
console.log(!!value2); // Output: true
console.log(!!value3); // Output: true
console.log(!!value4); // Output: true
Values such as non-zero numbers, non-empty strings, arrays, and objects are considered truthy in JavaScript, and thus are converted to true
when applied with !!
.
Ternary Operator
The ternary operator is a concise way to write an if-else statement.
const age = 20;
const canVote = age >= 18 ? "Yes" : "No";
console.log(canVote); // Yes
Using the Rest Parameter
function sum(...numbers) {
return numbers.reduce((accumulator, current) => accumulator + current, 0);
}
console.log(sum(1, 2, 3)); // 6
console.log(sum(4, 5, 6, 7)); // 22
console.log(sum(10)); // 10
function greet(first, ...rest) {
console.log(`Greet ${first}!`);
console.log('Greetings also to:', rest.join(', '));
}
greet('Antonio', 'Bob', 'Charlie', 'Dave');
// Output:
// Greet Antonio!
// Greetings also to: Bob, Charlie, Dave
Measure performance with console.time
console.time("test")
let value = 1;
for (let i = 0; i < 100_000_000; i++) {
value += i;
}
console.timeEnd("test")
Printing with console.table
const players = ["Mario", "Luigi", "Toad"];
const player = {
name: "Mario",
age : 25
}
console.table(player);
console.table(players);
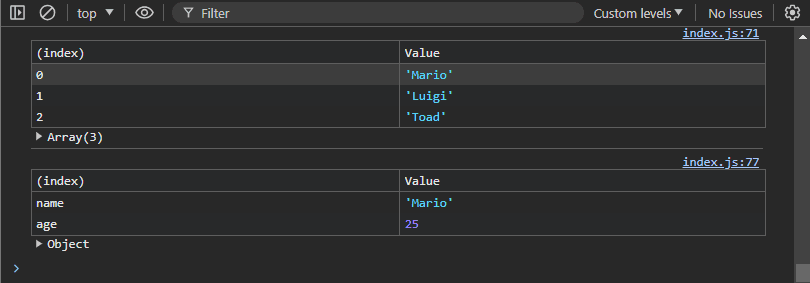
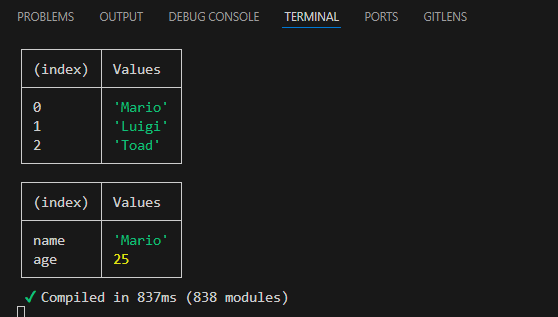
Styling the console.log
const styles = `padding: 50px;
background-color: blue;
color: white;
font-size:50px;
`;
console.log("%c Ciaoooooo !!!", styles);
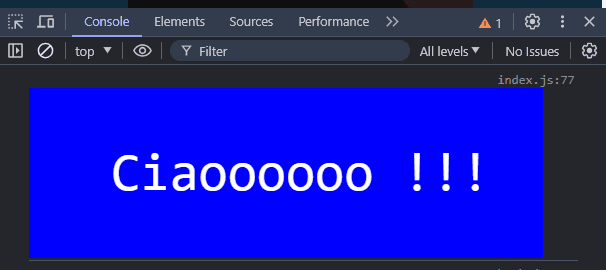