Practical Guide to Display Flex
Discover how to use Display Flex in CSS to create modern and responsive layouts.
Published:Introduction to Flexbox
Flexbox radically transforms how we organize and manage layouts on web pages. With display: flex
, it's possible to align and distribute elements within a container with ease, automatically adapting to any screen size. In this guide, we'll explore how to use display: flex
to design responsive and visually appealing layouts, supported by practical examples and tips.
Basic Concepts of Flexbox
When using Flexbox to create responsive layouts, it is important to know some fundamental concepts that govern the behavior of elements within the flexible container. These concepts will help you understand how elements align and distribute in the available space:
- Flex Container: The element that contains the flex items and to which
display: flex
is applied. - Flex Items: The elements within the flex container, arranged and aligned along the axes.
- Main Axis: The main axis along which the flex items align; it can be horizontal or vertical.
- Cross Axis: The axis perpendicular to the main axis, which handles cross alignment.
- Main Start/Main End: The start and end points of the main axis, defining the direction of alignment.
- Cross Start/Cross End: The start and end points of the secondary axis, for vertical or horizontal alignment.
- Main Size/Cross Size: The dimensions of the flex container along the main and secondary axes.
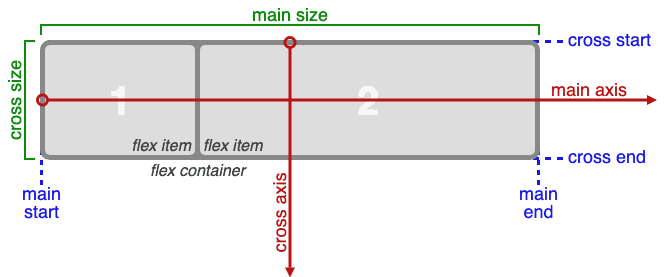
Parent Properties
display flex
The display: flex
property transforms an element into a flex container, altering how its children are arranged. Unlike the default behavior of a container with display: block
, where elements are arranged one below the other, with display: flex
the elements align horizontally but occupy only as much space as their content requires, unless otherwise specified with properties like flex-grow
.
.container {
display: flex;
}
display:
flex-direction
The flex-direction
property defines the main direction along which the child elements of a flex container are arranged. By default, elements align horizontally from left to right (direction row
), but this property offers various options to control the flow of elements.
The main options for flex-direction
are:
- row: Arranges elements horizontally from left to right (default).
- row-reverse: Arranges elements horizontally from right to left.
- column: Arranges elements vertically from top to bottom.
- column-reverse: Arranges elements vertically from bottom to top.
By using flex-direction
, you can easily change the orientation of elements based on layout needs, making the design more flexible and adaptable.
.container {
/* default value row */
display: flex;
flex-direction: row | row-reverse | column | column-reverse;
}
flex-direction:
flex-wrap
The flex-wrap
property controls whether the child elements of a flex container should be laid out on a single line or can wrap onto multiple lines when available space is insufficient. By default, all elements are arranged on a single line, but with flex-wrap
, you can modify this behavior.
The main options for flex-wrap
are:
- nowrap: Elements remain on a single line, ignoring available space (default).
- wrap: Elements wrap onto new lines if space on the main line is insufficient.
- wrap-reverse: Similar to
wrap
, but new lines are arranged in reverse order (from top to bottom).
Using flex-wrap
is useful when you want elements to dynamically adapt to containers of varying sizes, ensuring that the layout remains tidy and readable even in tight spaces.
.container {
display: flex;
flex-wrap: nowrap | wrap | wrap-reverse;
}
flex-wrap:
justify-content
The justify-content
property defines how to distribute free space along the main axis of the flex container and how to align children within it. It is particularly useful when you want to control the horizontal alignment of elements in a container with flex-direction: row
.
The main options for justify-content
are:
- flex-start: Elements are aligned at the start of the main axis (default).
- flex-end: Elements are aligned at the end of the main axis.
- center: Elements are centered along the main axis.
- space-between: Free space is evenly distributed between the elements, with the first and last elements aligned to the edges of the container.
- space-around: Free space is evenly distributed around the elements, creating equal spaces between each element and the container edges.
- space-evenly: Distributes space evenly among the elements, including the edges of the container.
Using justify-content
, you can precisely control the alignment of child elements, making the layout flexible and adaptable to different container widths.
.container {
display: flex;
justify-content: flex-start | flex-end | center | space-between | space-around | space-evenly;
}
justify-content:
align-items
The align-items
property controls the alignment of child elements along the cross axis (cross axis) of the flex container. It is useful for defining how elements are distributed vertically (when flex-direction
is set to row
) or horizontally (when flex-direction
is set to column
).
The main options for align-items
are:
- stretch: Elements stretch to fill the entire cross axis space (default).
- flex-start: Elements are aligned at the start of the cross axis.
- flex-end: Elements are aligned at the end of the cross axis.
- center: Elements are centered along the cross axis.
- baseline: Elements are aligned based on their text baseline.
With align-items
, you can control the vertical or horizontal alignment of elements based on the axis direction, improving the aesthetics and consistency of the layout.
.container {
display: flex;
align-items: stretch | flex-start | flex-end | center | baseline;
}
align-items:
2 item
4 item
align-content
The align-content
property manages the distribution of space between the rows of a flex container when there are multiple rows of elements. This property is relevant only when child elements wrap and occupy more than one row, for example, when using flex-wrap
.
The main options for align-content
are:
- stretch: Rows are stretched to fill the entire available space (default).
- flex-start: Rows are aligned at the start of the container.
- flex-end: Rows are aligned at the end of the container.
- center: Rows are centered within the available space.
- space-between: Free space is evenly distributed between the rows.
- space-around: Free space is evenly distributed around each row.
- space-evenly: Distributes space evenly among the rows, including the edges of the container.
Align-content
is useful for controlling the arrangement of rows within a container with multiple rows, improving vertical space management and the aesthetics of the layout.
.container {
display: flex;
flex-wrap: wrap;
align-content: stretch | flex-start | flex-end | center | space-between | space-around | space-evenly;
}
align-content:
gap
The gap
property (also known as grid-gap
in grid layouts) is used to define the space between child elements of a flex container. This property is particularly useful for adding margins between flex items without having to manually apply margins to each element.
With gap
, you can specify a uniform distance between elements, simplifying space management in the layout. It's possible to use a single value for a uniform space on both axes or two values to specify the space along the main and cross axes separately.
Examples of using gap
:
- gap: 10px; - Sets a space of 10px between all flex items.
- gap: 10px 20px; - Sets a space of 10px along the main axis and 20px along the cross axis.
.container {
display: flex;
flex-wrap: wrap;
gap: 10px;
gap: 10px 20px; /* row-gap column gap */
row-gap: 10px;
column-gap: 20px;
}
gap:
50pxChildren Properties
order
The order
property allows you to control the display order of flex items within a flex container. By default, all elements have an order
value of 0
, meaning they are displayed in the order they appear in the HTML code.
By changing the value of order
, you can alter the arrangement of elements independent of the order in which they are defined in the markup. Elements with a lower order
value will be displayed before those with a higher value.
.item-1 {
order: 1;
}
.item-2 {
order: 2;
}
align-self
The align-self
property allows you to modify the alignment of a single child element within a flex container, overriding the alignment defined by the container's align-items
property. This property is useful when you want a particular element to align differently from the others.
The main options for align-self
are the same as align-items
:
- auto: The element inherits the alignment defined by the container's
align-items
(default). - stretch: The element stretches to fill the entire available space along the cross axis.
- flex-start: The element is aligned at the start of the cross axis.
- flex-end: The element is aligned at the end of the cross axis.
- center: The element is centered along the cross axis.
- baseline: The element is aligned based on the text baseline.
.container {
display: flex;
align-items: center;
}
.item-3 {
align-self: stretch;
}
align-self:
flex-grow
The flex-grow
property determines how much a child element should grow relative to other elements within a flex container when there is available space. By default, all elements have a flex-grow
value of 0
, meaning they will not grow beyond their base size.
When a positive value is assigned to flex-grow
, the element will expand to occupy the available space in the container in proportion to the specified value. For example, an element with flex-grow: 2
will grow twice as much as an element with flex-grow: 1
.
Examples of using flex-grow
:
- flex-grow: 0; - The element does not grow, maintaining its base size (default).
- flex-grow: 1; - The element grows to occupy available space evenly with other elements that have the same value.
- flex-grow: 2; - The element grows to occupy twice the space compared to elements with
flex-grow: 1
.
.item-1 {
flex-grow: 2;
}
.item-2 {
flex-grow: 1;
}
.item-3 {
flex-grow: 0;
}
item 1 flex-grow:
item 2 flex-grow:
item 3 flex-grow:
flex-shrink
The flex-shrink
property controls a child element's ability to shrink within a flex container when space is limited. By default, all elements have a flex-shrink
value of 1
, meaning they will shrink in proportion to other elements to fit the available space.
A higher value of flex-shrink
will cause the element to shrink more than others, while a value of 0
will prevent the element from shrinking, maintaining its original size.
Examples of using flex-shrink
:
- flex-shrink: 0; - The element does not shrink, maintaining its base size.
- flex-shrink: 1; - The element shrinks in proportion to other elements (default).
- flex-shrink: 2; - The element shrinks twice as much as elements with
flex-shrink: 1
.
TIPS: Use flex-shrink: 0
on SVG elements to prevent them from shrinking when available space is limited.
.item-1 {
flex-shrink: 2;
}
.item-2 {
flex-shrink: 1;
}
.item-3 {
flex-shrink: 0;
}
item 1 flex-shrink:
item 2 flex-shrink:
item 3 flex-shrink:
flex-basis
The flex-basis
property defines the initial size of a child element within a flex container before any available space is distributed. This property establishes a baseline for the size of the element, which can be overridden by flex-grow
and flex-shrink
properties if necessary.
Flex-basis
can be expressed in various valid measurement units, such as pixels (px), percentages (%), or relative values (em, rem), and can also be set to specific keywords like auto
or intrinsic sizing keywords.
Examples of using flex-basis
:
- flex-basis: 100px; - The element will have an initial size of 100 pixels.
- flex-basis: 50%; - The element will initially occupy 50% of the available space in the container.
- flex-basis: auto; - The element will assume its natural size based on the content.
- flex-basis: max-content; - The element will have the size of the maximum content without wrapping.
- flex-basis: min-content; - The element will have the minimum size necessary to contain the content without cutting it off.
- flex-basis: fit-content; - The element will adjust to the content, with the minimum size possible that does not cause overflow.
Flex-basis
is useful for defining the base width or height of an element, providing a starting point for flexible and adaptable layout. This property allows you to control how elements are sized within a flex container, using specific units or content-based sizes.
.item-1 {
flex-basis: 300px;
}
item 1 flex-basis:
flex
The flex
property is a powerful shorthand that combines three fundamental properties of Flexbox: flex-grow
, flex-shrink
, and flex-basis
. With flex
, you can control with a single declaration how much a child element should grow, shrink, and what should be its initial size within a flex container.
The syntax for the flex
property is:
flex: <flex-grow> <flex-shrink> <flex-basis>;
Common examples of using flex
:
- flex: 1; - Shortcut for
flex: 1 1 0%
, where the element can grow and shrink based on available space, starting from a base size of 0. - flex: 0 1 auto; - The element can shrink but not grow, with a base size automatically determined by its content.
- flex: 2 1 100px; - The element grows twice as much as others, shrinks when necessary, and starts from an initial size of 100px.
Using the flex
property simplifies control over the flexible behavior of elements, as it allows you to combine the functionalities of flex-grow
, flex-shrink
, and flex-basis
into a single line of code.
.container {
display: flex;
}
.item-1 {
flex: 1 2 100px; /* flex-grow: 1; flex-shrink: 2; flex-basis: 100px */
}
.item-2 {
flex: 2 1 200px; /* flex-grow: 2; flex-shrink: 1; flex-basis: 200px */
}
.item-3 {
flex: 1 1 150px; /* flex-grow: 1; flex-shrink: 1; flex-basis: 150px */
}
display: